I was looking at a reddit post recently where someone had posted this picture
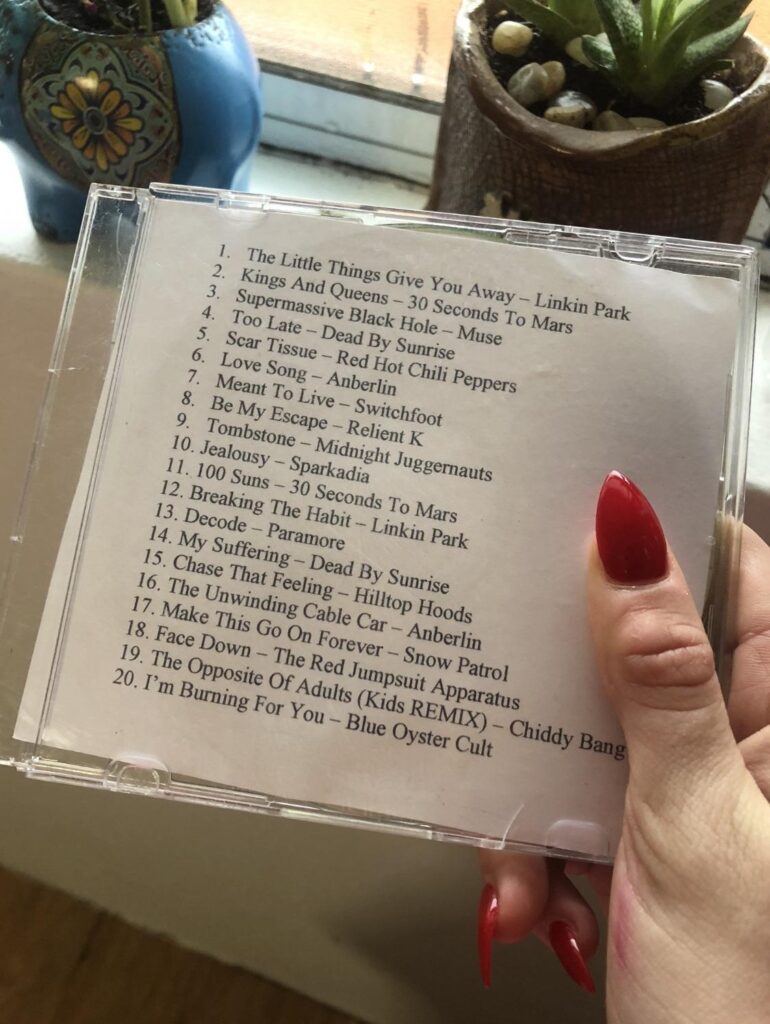
The person had described that it was a mixtape made by her first boyfriend in 2008. Here’s the original post
I like many of the songs in this list and was curious to listen to the ones that I am not familiar with. I wanted to add all of these songs to a separate playlist and listen to them on my next run. I come across this situation often. Last week, I was looking for songs similar to ‘Nothing’s Gonna Hurt You Baby’ on reddit. I wanted to add multiple songs from this page to a Spotify playlist but had no easy way of doing so. This is my most favorite way to discover new music and I felt a python program to bulk add songs to a Spotify playlist would be useful for me. Writing such a program was easier than I thought. I’m sure the program would be useful for many people like me. You can modify it add hundreds of songs from a spreadsheet, read songs from your offline collection and add them to Spotify playlist, etc.
Here’s how I did it in like 10 minutes using Python and an amazing library called Spotipy.
I first ran the image through an OCR app online to get the list of songs in simple text. Then I put the list of songs in a python list called ‘songs’ so that I can iterate over them in a python program. Here’s the songs python list I made from the above image.
songs = [
"The Little Things Give You Away - Linkin Park",
"Kings And Queens - 30 Seconds To Mars",
"Supermassive Black Hole - Muse",
"Too Late - Dead By Sunrise",
"Scar Tissue - Red Hot Chili Peppers",
"Love Song - Anberlin",
"Meant To Live - Switchfoot",
"Be My Escape - Relient K",
"Tombstone - Midnight Juggernauts",
"Jealousy - Sparkadia",
"100 Suns - 30 Seconds To Mars",
"Breaking The Habit - Linkin Park",
"Decode - Paramore",
"My Suffering - Dead By Sunrise",
"Chase That Feeling - Hilltop Hoods",
"The Unwinding Cable Car - Anberlin",
"Make This Go On Forever - Snow Patrol",
"Face Down - The Red Jumpsuit Apparatus",
"The Opposite Of Adults (Kids REMIX) - Chiddy Bang",
"I'm Burning For You - Blue Oyster Cult"
]
I created a virtual environment on my project directory, activated it and installed spotipy using pip3
pip3 install spotipy
I went to Spotify Developer site dashboard (https://developer.spotify.com/dashboard) and created a new app there. I just gave https://localhost/ as the ‘Redirect URI’ and gave a basic description for creating the app since they are mandatory fields.
I copied the client ID and client secret from the app’s settings. Then I wrote the below code to help me create a new Spotify playlist with the songs above:
import spotipy
from spotipy.oauth2 import SpotifyOAuth
# Set up your Spotify Developer credentials
SPOTIPY_CLIENT_ID = 'CLIENT-ID'
SPOTIPY_CLIENT_SECRET = 'CLIENT-SECRET'
SPOTIPY_REDIRECT_URI = 'http://localhost/'
# Songs list
songs = [
"The Little Things Give You Away - Linkin Park",
"Kings And Queens - 30 Seconds To Mars",
"Supermassive Black Hole - Muse",
"Too Late - Dead By Sunrise",
"Scar Tissue - Red Hot Chili Peppers",
"Love Song - Anberlin",
"Meant To Live - Switchfoot",
"Be My Escape - Relient K",
"Tombstone - Midnight Juggernauts",
"Jealousy - Sparkadia",
"100 Suns - 30 Seconds To Mars",
"Breaking The Habit - Linkin Park",
"Decode - Paramore",
"My Suffering - Dead By Sunrise",
"Chase That Feeling - Hilltop Hoods",
"The Unwinding Cable Car - Anberlin",
"Make This Go On Forever - Snow Patrol",
"Face Down - The Red Jumpsuit Apparatus",
"The Opposite Of Adults (Kids REMIX) - Chiddy Bang",
"I'm Burning For You - Blue Oyster Cult"
]
# Authentication
sp = spotipy.Spotify(auth_manager=SpotifyOAuth(
client_id=SPOTIPY_CLIENT_ID,
client_secret=SPOTIPY_CLIENT_SECRET,
redirect_uri=SPOTIPY_REDIRECT_URI,
scope="playlist-modify-public" # This scope allows adding tracks to a playlist
))
# Get user's username
username = sp.me()['id']
# Create a new playlist
playlist = sp.user_playlist_create(user=username, name="PLAYLIST NAME")
# Search for tracks and add them to the playlist
track_ids = []
for song in songs:
result = sp.search(q=song, limit=1)
if result['tracks']['items']:
track_ids.append(result['tracks']['items'][0]['id'])
sp.user_playlist_add_tracks(user=username, playlist_id=playlist['id'], tracks=track_ids)
print(f"Added {len(track_ids)} songs to the playlist!")
When I ran this app using command python3 app.py, my browser opened the Spotify authorization page asking me to authorize the app. When I clicked on ‘Authorize’, I was redirected to a localhost URL. I copied this URL and paste it in my terminal for completing the auth request. That was it! All the 20 songs were added to a newly created Spotify playlist.
I hope you found this post useful. I would love it if you could leave a comment below 🙂
Leave a Reply