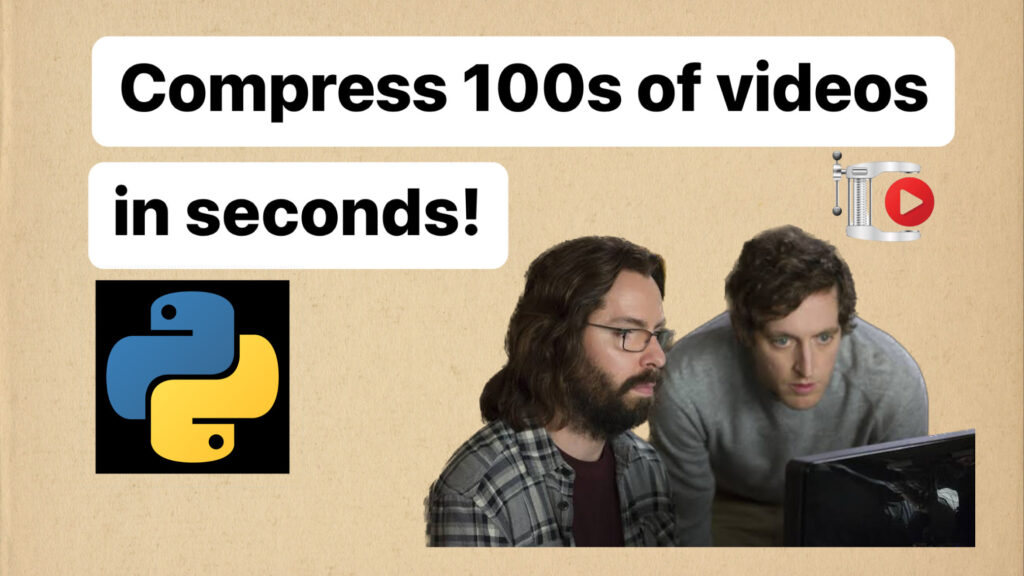
Why compress videos?
With the rise in digital videos, it is easier than ever to capture and store precious memories in our personal devices. However, storing these videos can take up a lot of space on our devices, which can lead to slow performance and other issues. Compressing videos will help you by
- Saving disk space
- Fastening uploads and downloads
- Improving performance
How to compress videos?
You’ve probably used an online tool like clideo.com or freeconvert.com to compress your videos. I’m going to show you how to use python and ffmpeg to convert the videos right on your desktop. This would be a self-hosted and free alternative to clideo.com and freeconvert.com for processing multiple videos at once.
What is ffmpeg?
FFmpeg is a free and open-source software project that produces libraries and programs for handling multimedia data. It includes a set of tools for handling video, audio, and other multimedia files and streams, including transcoding, streaming, and editing. It is often used in combination with other software, such as Python, to automate video processing tasks, like compressing all videos in a folder.
How to install ffmpeg?
To install FFmpeg, you can follow these steps:
- Go to the FFmpeg download page and select your operating system.
- Download the latest version of FFmpeg for your system.
- Extract the downloaded file to a directory of your choice.
- Add the FFmpeg executable to your system’s PATH environment variable so that you can run it from any directory.
After completing these steps, you should be able to use FFmpeg from the command line or in combination with other software, like Python, to compress videos in a folder.
How to compress videos using ffmpeg?
I normally use the following ffmpeg commanad in my terminal to compress videos individually.
ffmpeg -i input.mov -c:v libx264 -c:a copy -crf 20 output.mov
How to compress multiple videos?
You would need to use python along with ffmpeg to compress multiple videos. Python can be used to loop through multiple videos and run the above ffmpeg command on each of them.
How to use python and ffmpeg to compress multiple videos?
You need 2 python built-in libraries for this purpose – os and subprocess.
Subprocess module helps you run the ffmpeg command on all the videos that you loop through.
Let’s create a function called ‘compress_videos’ to loop through all the videos in a particular folder. Then we use subprocess to run the ffmpeg command inside the function to compress every video.
We are using a conditional that checks if the file we are looping through is a video before we run the ffmpeg command on it – this would prevent the program running into unnecessary errors.
We then assign the folder path to a variable and then call the compress_videos function on this variable.
Here’s the code:
import os
import subprocess
def compress_videos(folder_path):
for filename in os.listdir(folder_path):
if filename.endswith('.mov') or filename.endswith('.mp4'):
input_path = os.path.join(folder_path, filename)
output_path = os.path.join(folder_path, f'compressed_{filename}' )
ffmpeg_cmd = f'ffmpeg -i {input_path} -c:v libx264 -c:a copy -crf 20 {output_path}'
subprocess.run(ffmpeg_cmd, shell=True)
folder_path = 'FOLDER_PATH_HERE'
compress_videos(folder_path)
Here’s my video walk-through explaining the code and a short demo:
Leave a Reply