Why split an .mp3 file in the first place? Some of the common reasons:
Easier Navigation: Dividing a lengthy audio file into smaller sections makes it simpler to navigate, allowing users to quickly find and listen to specific parts without having to manually search through the entire file.
Better Organization: Breaking down a long file into smaller, topic-based segments can help users better understand and organize the content. This is particularly helpful for educational materials, podcasts, and audiobooks.
Easy to Share: Smaller files are easier to share, store, and transfer. Imagine putting up a 10GB .mp3 file on your website for people to download. People might be interested in a particular section of that file but you are giving them no other way but for them to download the entire thing. You would be doing them a favor and also save lot of resources by breaking down the file into multiple parts and allowing them to choose which ones they want to download.
Compatibility: Some older devices or software might have limitations on the file size they can handle, so splitting a large .mp3 file into smaller segments can ensure compatibility with a wider range of devices and applications.
Error recovery: If a file becomes corrupted or damaged (happens way more often than you can imagine), having multiple smaller parts can help minimize data loss, as only the affected part may need to be replaced or repaired.
How do we break an .mp3 into parts?
If you only have a single .mp3 file that you want to break into parts, you don’t really need python to do it, however long it could be. All you need it ffmpeg.
Open you terminal, navigate to the folder with the original .mp3 file and run the following command
ffmpeg -i input.mp3 -map 0 -f segment -segment_time 1800 -c copy out%03d.mp3
‘input’ is the name of the original .mp3 file and 1800 is the number of seconds you want every chunk’s length to be. For example, if you run this command on a 10 hour .mp3 file, you would get 20 .mp3 files all 30 minutes long (1800 seconds = 30 minutes). If the original file was 5 hours and 13 minutes long, this command would give you 11 parts. The first 10 parts would all be 30 minutes long and the last part would be 13 minutes long.
You can change the 1800 in the command to any number of seconds as you would like.
Let me show you an example. Here’s an .mp3 file in a folder called mp3 on my desktop. It is a Lex Fridman podcast episode that I downloaded from his website that is more than 5 hours long.
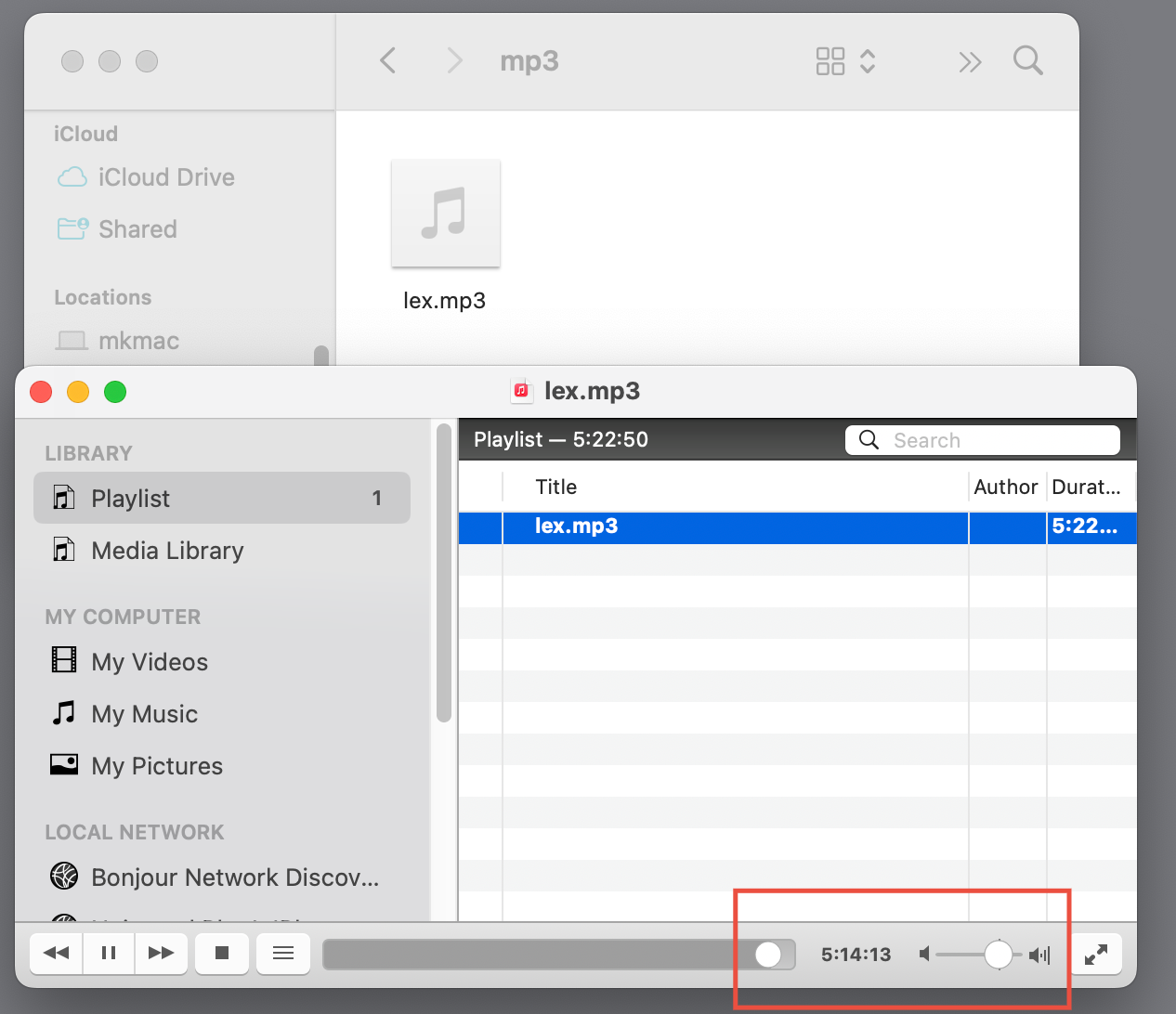
Let me show you how I navigate to this folder and run the above ffmpeg command there. I am changing input.mp3 to lex.mp3 which is the actual filename.

The above ffmpeg command generated 11 .mp3 files – 10 of them were 30 minutes long and the last one was about 21 minutes.
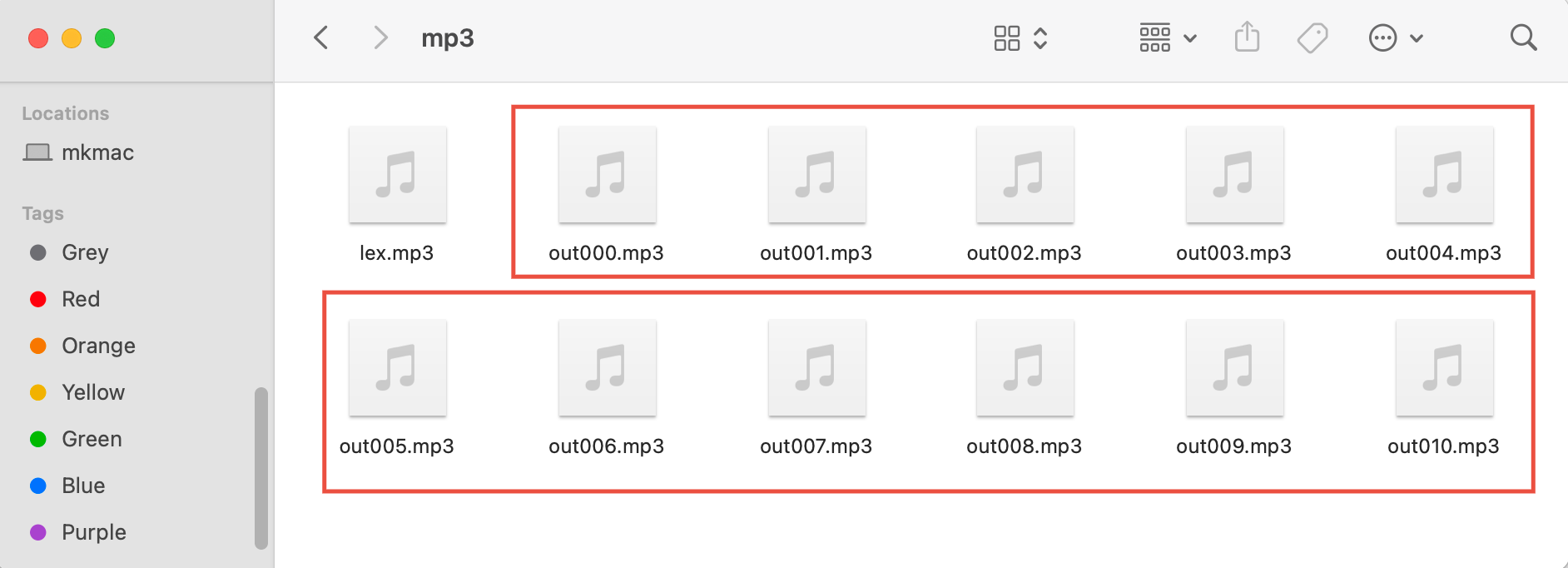
Now, what do we do when we have a folder full of long .mp3 files like lex.mp3 above and we want to break them all down, put the parts into separate folders for better organization, etc.? That is when we need python.
How to use python to break multiple .mp3 files into parts?
Now I have 3 such podcast episodes in the folder. 1 episode from ‘The American Life’, 1 from ‘The Tim Ferriss Show’ and 1 from Lex Fridman.
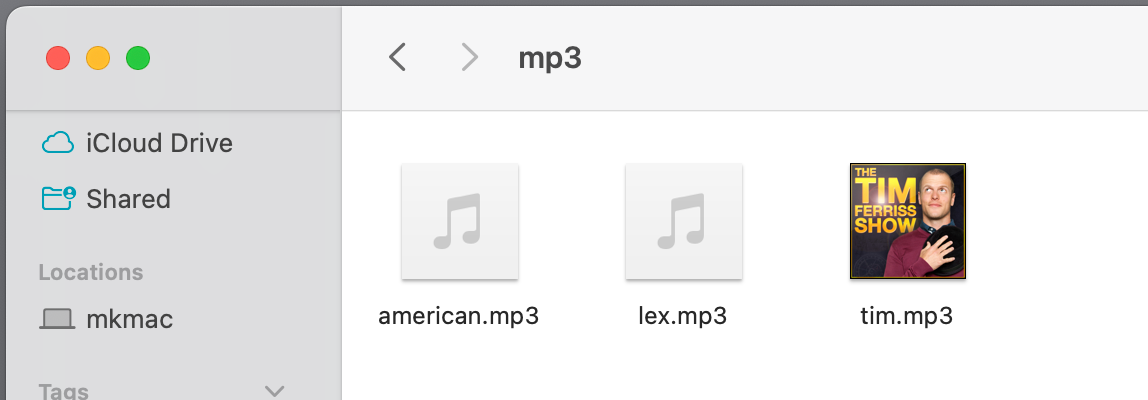
I want to break each of these episodes into 30 minute chunks and arrange them in folders. For eg., I want a folder called ‘lex’ that would contain all of the parts of the lex.mp3 file and so on.
We are going to use the same ffmpeg command that I have used earlier but we are going to run it through a loop. We are going to make use of python’s inbuilt modules os and subprocess. os would help us access the files in a specified directory and subprocess would help us run Linux commands directly in a python script.
import os
import subprocess
Now we tell python where the long (original) .mp3 files are – we also specify the folder where we want the new folders with the parts to be. My original files are in a folder called ‘mp3’ on the desktop. I want the new folders to be in a folder called ‘new_mp3’ on the desktop.
source_folder = '/Users/muthu/Desktop/mp3'
output_base_folder = '/Users/muthu/Desktop/new_mp3'
We then tell python to loop through all the .mp3 files alone in the source folder. We also specify how to name the output files.
for file_name in os.listdir(source_folder):
if file_name.endswith('.mp3'):
# Create an output folder for the current file
output_folder = os.path.join(output_base_folder, os.path.splitext(file_name)[0])
if not os.path.exists(output_folder):
os.makedirs(output_folder)
# Construct the input and output file paths
input_file = os.path.join(source_folder, file_name)
output_file = os.path.join(output_folder, 'out%03d.mp3')
We now make the ‘input’ and ‘output’ in the original ffmpeg command dynamic using f strings and then call the command using subprocess.run
ffmpeg_command = f'ffmpeg -i {input_file} -map 0 -f segment -segment_time 1800 -c copy {output_file}'
subprocess.run(ffmpeg_command, shell=True)
That is it! I save this program as ‘app.py’ inside the mp3 file and run it from the terminal.

I now have a folder called ‘new_mp3’ with 3 folders – each containing the parts of the podcasts neatly arranged with proper filenames.
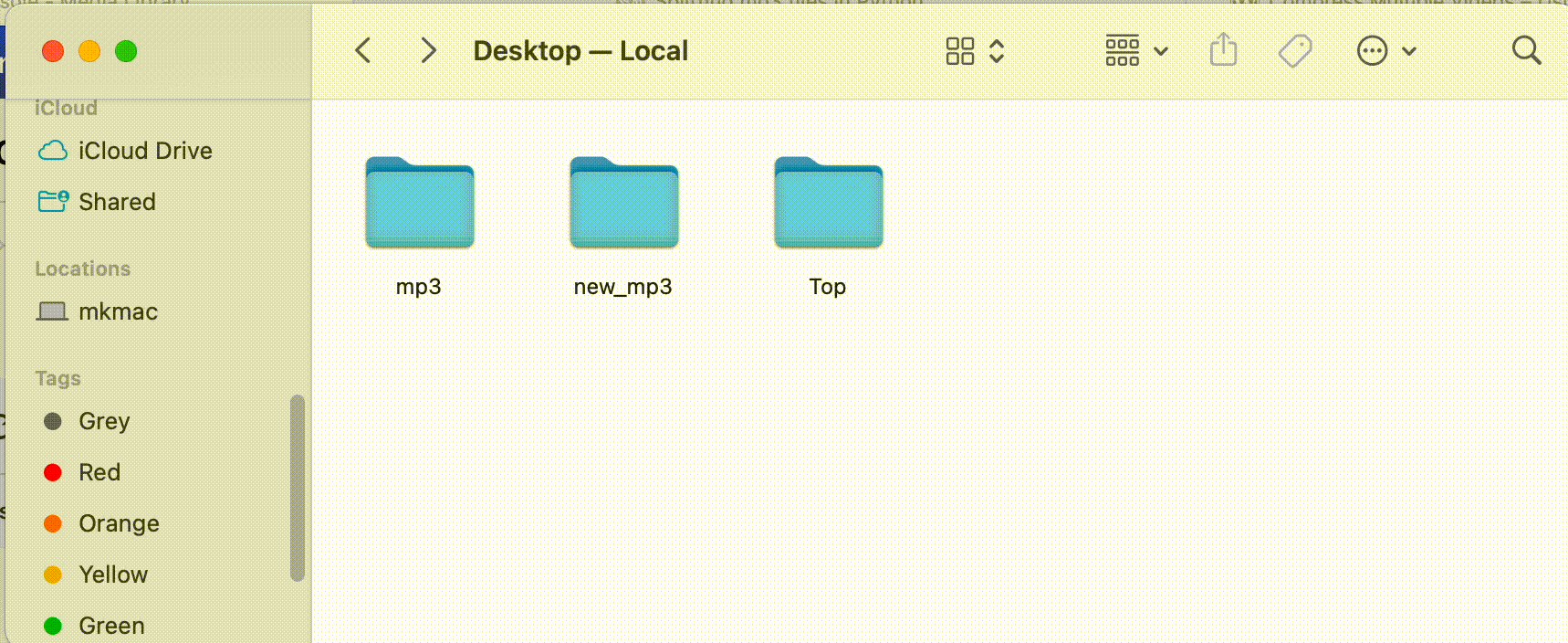
Here’s the entire code in my app.py file for you to play around:
import os
import subprocess
#remember to change these values to your own folder paths
source_folder = 'PATH'
output_base_folder = 'PATH'
for file_name in os.listdir(source_folder):
if file_name.endswith('.mp3'):
# Create an output folder for the current file
output_folder = os.path.join(output_base_folder, os.path.splitext(file_name)[0])
if not os.path.exists(output_folder):
os.makedirs(output_folder)
# Construct the input and output file paths
input_file = os.path.join(source_folder, file_name)
output_file = os.path.join(output_folder, 'out%03d.mp3')
ffmpeg_command = f'ffmpeg -i {input_file} -map 0 -f segment -segment_time 1800 -c copy {output_file}'
subprocess.run(ffmpeg_command, shell=True)
Here’s a video walk-through:
Leave a Reply